During the New Year’s party of our college, all the members of LYM lab put on an exceptional dance performance. The performance was full of energy and excitement, and it was evident that each member had put in a lot of effort and time to make it happen. Our performance was a great source of amusement for many students and teachers alike, and it brought New Year’s greetings to everyone. The reason our performance was fantastic was because of the amount of practice and hard work that each member had put in. We spent countless hours in the lab, rehearsing and perfecting every move to make sure that our performance was flawless. Our hard work paid off as our performance was seamless, and we were able to bring joy and happiness to everyone watching. The performance brought all of us lab members closer together and made us more familiar with each other. It was an incredible experience to work together and achieve something as a team. The joy and sense of accomplishment that we felt after our performance was indescribable.
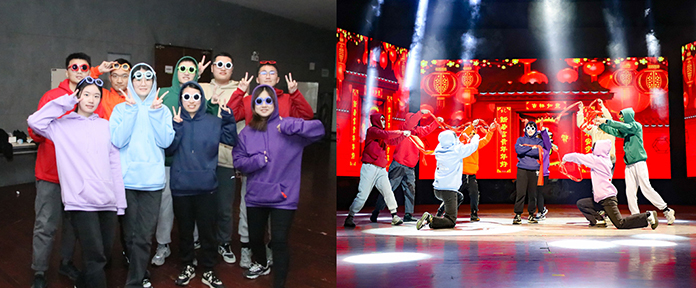
As time has unfolded, we have journeyed through a fulfilling and challenging year together in 2023. Let us now come together to share the harvests and joys of this past year and collectively gaze into the boundless possibilities that lie ahead.
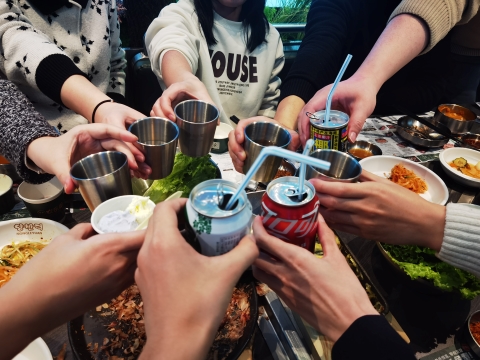
Our professor’s paper titled “Multimodal Epigenetic Sequencing Analysis (MESA) of Cell-Free DNA for Non-Invasive Colorectal Cancer Detection” was accepted by Genome Medicine. The paper was recognized for its contributions to the field of non-invasive cancer detection.
Today was a truly special day at our lab! Prof. Jingyi Jessica Li joined us for lunch, and we sampled the delicious flavors of Suzhou cuisine. We chatted, laughed, and shared stories and updates about our research. Professor Li’s warm personality and engaging stories made the lunch even more enjoyable. After lunch, we invited Prof. Li to visit our lab, and briefly introduced our work environment.
Thank you, Prof. Li, for taking the time to visit us and share your wisdom. Your visit was truly a highlight of our lab’s journey, and we are all grateful for the opportunity to learn from you!
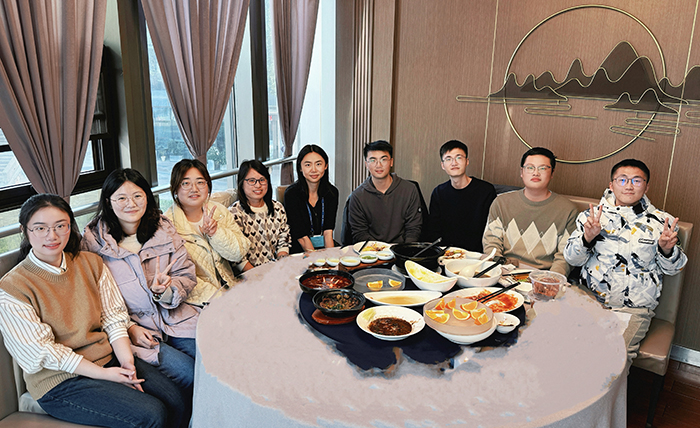
Recently, our lab was delighted to host a visit from Prof. Yu Li. We took the opportunity to share our progress and challenges of bioinformatics research. Professor Li, in turn, introduced his own research and provided valuable insights and perspectives that expanded our understanding of the field.
Lunch was a highlight of the visit, serving as a break from our work and providing an opportunity for casual conversation. We discussed various topics, ranging from research to daily life, and everyone felt refreshed, inspired, and enriched. Prof. Li’s visit was a fruitful and enjoyable experience. It fostered collaborations, expanded our horizons, and left us feeling happy and grateful for the opportunity to learn from such an esteemed scholar. We look forward to future opportunities to continue this exchange of ideas and experiences!